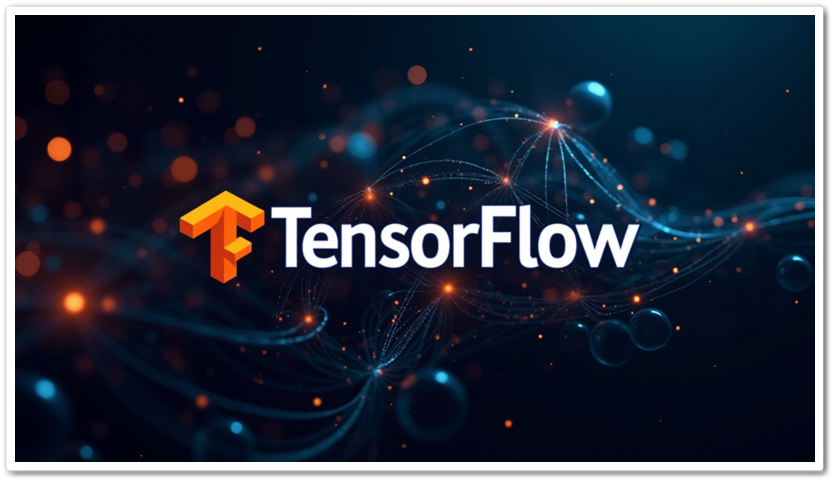
What is TensorFlow?
TensorFlow is an open-source machine learning (ML) framework developed by Google. Originally released in 2015, it has since become one of the most widely used tools for deep learning (DL) and artificial intelligence (AI) research and development. TensorFlow provides a flexible ecosystem for building and deploying machine learning models across a variety of platforms, including CPUs, GPUs, and TPUs. The name “TensorFlow” is derived from its ability to process tensors (multi-dimensional arrays) and its computation graph execution model.
Key Features of TensorFlow
TensorFlow offers several advantages that make it a powerful choice for machine learning practitioners:
Flexibility and Scalability
-
TensorFlow supports both high-level and low-level APIs, making it suitable for researchers and engineers alike.
-
It enables deployment across different environments, from mobile devices to cloud-based infrastructures.
-
Supports both eager execution (imperative programming) and graph execution (declarative programming).
Multi-Platform Support
-
Can be executed on various devices, including desktops, servers, mobile devices (Android/iOS), and embedded systems.
-
Offers native integration with TensorFlow Lite for mobile applications and TensorFlow.js for web-based machine learning.
-
TensorFlow Extended (TFX) provides end-to-end solutions for deploying machine learning pipelines in production.
Rich API Support
-
Provides comprehensive support for multiple programming languages, including Python, C++, Java, and JavaScript.
-
High-level APIs such as Keras simplify the process of building deep learning models.
-
Low-level APIs allow for custom operations and optimizations for advanced users.
GPU and TPU Acceleration
-
TensorFlow efficiently utilizes hardware acceleration, making it ideal for large-scale computations.
-
Supports CUDA-enabled GPUs for training models faster than on traditional CPUs.
-
Google’s custom Tensor Processing Units (TPUs) provide specialized hardware acceleration for deep learning tasks.
Strong Community and Ecosystem
-
Backed by Google and widely adopted in both academia and industry.
-
Extensive documentation and tutorials available on TensorFlow’s official website (https://www.tensorflow.org/).
-
Large and active open-source community contributing to enhancements, bug fixes, and new features.
-
Compatible with various ML and AI tools such as TensorBoard for visualization, TF Agents for reinforcement learning, and TF Probability for probabilistic modeling.
Applications of TensorFlow
TensorFlow is used in a variety of domains due to its powerful capabilities. Some common use cases include:
-
Deep Learning Model Development:
-
Implementing architectures like Convolutional Neural Networks (CNNs), Recurrent Neural Networks (RNNs), Transformers, and Generative Adversarial Networks (GANs).
-
-
Natural Language Processing (NLP):
-
Sentiment analysis, text classification, machine translation, chatbots, and speech recognition.
-
-
Computer Vision:
-
Image classification, object detection, facial recognition, and style transfer.
-
-
Reinforcement Learning:
-
Training AI agents for decision-making tasks in robotics, gaming (e.g., AlphaGo), and automation.
-
-
Time-Series Analysis and Predictive Modeling:
-
Used in finance, healthcare, marketing, and various other industries for forecasting and anomaly detection.
-
-
Healthcare and Medical AI:
-
Diagnosing diseases, medical image analysis, drug discovery, and patient monitoring systems.
-
Installing and Using TensorFlow
TensorFlow can be easily installed using pip, the Python package manager.
pip install tensorflow
A simple example to perform basic tensor operations using TensorFlow:
import tensorflow as tf
# Creating two tensors
a = tf.constant(5)
b = tf.constant(3)
# Performing a simple operation
c = a + b
print(c.numpy()) # Output: 8
Building a Neural Network with TensorFlow and Keras
TensorFlow includes Keras, a high-level API that simplifies the creation of deep learning models.
from tensorflow import keras
from tensorflow.keras import layers
# Defining a simple neural network model
model = keras.Sequential([
layers.Dense(64, activation='relu', input_shape=(10,)),
layers.Dense(32, activation='relu'),
layers.Dense(10, activation='softmax')
])
# Compiling the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Summary of the model architecture
model.summary()
Advanced TensorFlow Features
TensorBoard – Visualization Tool
TensorBoard is TensorFlow’s visualization toolkit for tracking training progress, analyzing performance metrics, and visualizing model architectures.
import tensorflow as tf
# Load TensorBoard extension
%load_ext tensorboard
# Create a log directory
log_dir = "logs/fit/"
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir=log_dir, histogram_freq=1)
To start TensorBoard, run:
tensorboard --logdir=logs/fit
TensorFlow Datasets
TensorFlow Datasets (TFDS) provides a collection of ready-to-use datasets for ML research.
import tensorflow_datasets as tfds
dataset, info = tfds.load("mnist", as_supervised=True, with_info=True)
print(info)
TensorFlow Lite – Deploying Models on Edge Devices
TensorFlow Lite allows ML models to run efficiently on mobile and edge devices.
converter = tf.lite.TFLiteConverter.from_saved_model("model_path")
tflite_model = converter.convert()
Conclusion
TensorFlow is a versatile and powerful machine learning framework widely used for deep learning and AI applications. With support for multiple platforms, hardware accelerators, and a robust ecosystem of tools, it remains a top choice for both researchers and industry professionals. Whether you are just starting with ML or working on large-scale AI projects, TensorFlow provides the necessary tools and flexibility to meet your needs.
To dive deeper into TensorFlow, visit the official website: TensorFlow.org and explore tutorials, API references, and community resources.
[…] TensorFlow: An Open-Source Machine Learning Framework by Google […]
[…] TensorFlow: An Open-Source Machine Learning Framework by Google […]